Introduction to SmartGWT
I recently started working with
SmartGWT, a framework based on
GWT that provides a comprehensive widget library for your application UI as well as assistance for server-side for data management. You can take look at its pretty functionality at the
SmartGWT showcase.
Creating the GWT project
First step is to download the library from the
downloads section. The version I will be using for this tutorial is 2.1 (download directly from
here). Extract the ZIP file and in the new directory you will find the framework's documentation, some samples, “Hello World” examples and of course the necessary JAR files. Open the
JavaDocs in a new browser tab.
Next we create a new “Web Application Project” in Eclipse. Choose the profound name “SmartGWTIntroProject” for the project and the appropriate packaging. Make sure that the “Use Google Web Toolkt” checkbox is checked (Google's App Engine is not needed, so do not select that). The wizard will look something like this:
Adding the SmartGWT library
After the project skeleton is created, browse through your filesystem to the project's location and create a new folder named “lib”. Copy the “smartgwt.jar” file from the extracted ZIP to the newly created folder and refresh the project in Eclipse, so that the new file appears there. Then configure your project's classpath to include the JAR file (Project → Properties → Java Build Path → Add JARs...). Standard stuff so far. The expanded project in Eclipse should look like this:
Then edit the module xml file (named “SmartGWTIntroProject.gwt.xml”) and add the following line after the standard “inherits” declarations:
1 | < inherits name = "com.smartgwt.SmartGwt" /> |
The module xml file will be:
01 | <? xml version = "1.0" encoding = "UTF-8" ?> |
02 | < module rename-to = 'smartgwtintroproject' > |
04 | < inherits name = 'com.google.gwt.user.User' /> |
15 | < inherits name = "com.smartgwt.SmartGwt" /> |
18 | < entry-point class = 'com.javacodegeeks.smartgwt.client.client.SmartGWTIntroProject' /> |
21 | < source path = 'client' /> |
22 | < source path = 'shared' /> |
This allows GWT to know that your application will be using the SmartGWT library.
UPDATE: The 'com.google.gwt.user.theme.standard.Standard' declaration should be removed or commented out like above, since it conflicts with certain SmartGWT styles.
After this, locate the main HTML inside the “war” folder. Edit that and add the following line before the compiled module declaration :
1 | < script >var isomorphicDir="smartgwtintroproject/sc/";</ script > |
UPDATE: From version 2.2, there is no longer need to define the isomorpihcDir value. Check the
Release Notes for Smart GWT 2.2. However, for this tutorial (version 2.1 used) the declaration is needed.
In the same file, scroll down and find the following lines:
1 | < td id = "nameFieldContainer" ></ td > |
2 | < td id = "sendButtonContainer" ></ td > |
Replace those with the following:
1 | < td id = "formContainer" ></ td > |
2 | < td id = "buttonContainer" ></ td > |
Those are the HTML elements that will hold the text item and the button that we will add later.
The full HTML file follows:
10 | < meta http-equiv = "content-type" content = "text/html; charset=UTF-8" > |
15 | < link type = "text/css" rel = "stylesheet" href = "SmartGWTIntroProject.css" > |
20 | < title >Web Application Starter Project</ title > |
27 | < script >var isomorphicDir="smartgwtintroproject/sc/";</ script > //add this line in own project |
28 | < script type = "text/javascript" language = "javascript" src = "smartgwtintroproject/smartgwtintroproject.nocache.js" ></ script > |
39 | < iframe src = "javascript:''" id = "__gwt_historyFrame" tabIndex = '-1' style = "position:absolute;width:0;height:0;border:0" ></ iframe > |
43 | < div style = "width: 22em; position: absolute; left: 50%; margin-left: -11em; color: red; background-color: white; border: 1px solid red; padding: 4px; font-family: sans-serif" > |
45 | Your web browser must have JavaScript enabled |
46 | in order for this application to display correctly. |
52 | Web Application Starter Project</ h1 > |
56 | < td colspan = "2" style = "font-weight:bold;" >Please enter your name:</ td > |
60 | < td id = "formContainer" ></ td > |
61 | < td id = "buttonContainer" ></ td > |
65 | < td colspan = "2" style = "color:red;" id = "errorLabelContainer" ></ td > |
71 | </ html > | | Creating the application entrypoint
When a GWT is created via Eclipse, a lot of auto-generated files are created. One of those is the main Java file in the “client” package, which works as the application's entrypoint. So, remove the generated code and add the following:
01 | package com.javacodegeeks.smartgwt.client.client; |
03 | import com.google.gwt.core.client.EntryPoint; |
04 | import com.google.gwt.user.client.ui.RootPanel; |
05 | import com.smartgwt.client.util.SC; |
06 | import com.smartgwt.client.widgets.IButton; |
07 | import com.smartgwt.client.widgets.events.ClickEvent; |
08 | import com.smartgwt.client.widgets.events.ClickHandler; |
09 | import com.smartgwt.client.widgets.form.DynamicForm; |
10 | import com.smartgwt.client.widgets.form.fields.TextItem; |
12 | public class SmartGWTIntroProject implements EntryPoint { |
14 | public void onModuleLoad() { |
16 | final DynamicForm form = new DynamicForm(); |
17 | final TextItem textItem = new TextItem(); |
18 | textItem.setTitle( "Name" ); |
19 | form.setFields(textItem); |
20 | final IButton button = new IButton( "Hello" ); |
22 | button.addClickHandler( new ClickHandler() { |
23 | public void onClick(ClickEvent event) { |
24 | String name = textItem.getValue().toString(); |
25 | SC.say( "Hello " + name); |
29 | RootPanel.get( "formContainer" ).add(form); |
30 | RootPanel.get( "buttonContainer" ).add(button); |
Make sure that the imported packages are as shown above, because SmartGWT uses classes with names same with those of the core GWT framework.
Launching the application
Next, we are ready to launch our application. Choose Run → Run As → Web Application and use your favourite browser to access the provided URL:
http://127.0.0.1:8888/SmartGWTIntroProject.html?gwt.codesvr=127.0.0.1:9997
You should be able to see the following:
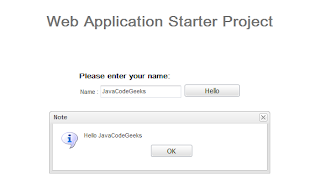
That's it. Now you are ready to create some cool applications, powered by SmartGWT.
|
Hello, I have a doubt, at the subject "Creating a GWT project", when you say
ReplyDelete" and the appropriate packaging", what exactly have I to put there?.
Which is the appropriate packaging?
Thanks